Associare un delegato tramite reflection
In questo esempio viene mostrato come collegarsi ad un delegato utilizzando reflection.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading;
namespace ConnectToADelegateUsingReflection
{
class Program
{
static void Main(string[] args)
{
MyEventGenerator myEventGenerator = new MyEventGenerator();
EventInfo myEventInfo = typeof(MyEventGenerator).GetEvent("MyEvent");
MethodInfo methodInfo = typeof(Program).GetMethod("OnMyEvent",
BindingFlags.NonPublic | BindingFlags.Static);
Delegate @delegate = Delegate.CreateDelegate(
myEventInfo.EventHandlerType, methodInfo);
myEventInfo.GetAddMethod()
.Invoke(myEventGenerator, new Object[] { @delegate });
Console.WriteLine("Press ENTER to exit");
Console.ReadLine();
}
static void OnMyEvent(Object sender, EventArgs e)
{
Console.WriteLine("MyEvent received");
}
}
}
|
|
A series of examples that show how to pass complex data (structures or arrays) from C# to C:
PassingStructArrayBetweenCandCSharp.h
#define DLL_EXPORT __declspec(dllexport)
#pragma pack(1)
typedef struct {
int X;
int Y;
int Z;
int MyFixedArray[10];
} MyComplexType;
extern "C"
{
DLL_EXPORT void __cdecl MyFunction2(MyComplexType* myComplexType);
}
PassingStructArrayBetweenCandCSharp.cpp
#include "stdafx.h"
#include "PassingStructArrayBetweenCandCSharp.h"
#include <stdio.h></stdio.h>
void MyFunction2(MyComplexType* myComplexType)
{
myComplexType->X = 100;
myComplexType->Y = 200;
myComplexType->Z = 300;
for (int i = 0; i < 10; i++) {
myComplexType->MyFixedArray[i] = i * 5;
}
}
|
|
Add-Ins is an application framework that can be used to create modular applications through the use of additional and extensible components.
In short, an application built using Add-Ins framework (MAF) is composed of a "host" and the add-ons, each add-on may be loaded in a separate application domain in order to ensure a certain level of isolation and therefore safety.
The communication between components is effected thus among different application domains and it is based on .NET Remoting, this feature requires the use of contracts or types serializable by channel remoting used so as to pass through the isolation boundary.
The programming model is implemented through the use of namespace:
- System.AddIn,
- System.AddIn.Hosting,
- System.AddIn.Pipeline,
- System.AddIn.Contract.
Below this is a project that briefly shows how you can use MAF to create an application based on add-ins, to make it more understandable and visible use of the add-ins framework I decided to create the add-ins which make up the user interface of an application.
UsingMAFWindowsForms.zip
|
|
How to create WCF service over Mono Framework
|
|
Come caricare un file XAML a tempo di esecuzione ed inserirlo in una finestra
<Page xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300"
Title="Page1">
<Grid>
<DockPanel HorizontalAlignment="Stretch">
<Button Name="button1" Content="Button" Height="100" Margin="10,10,10,10" />
</DockPanel>
</Grid>
</Page>
Per caricare lo XAML visuailizzato sopra:
//...
StreamReader mysr = new StreamReader("Page1.xaml");
DependencyObject rootObject = XamlReader.Load(mysr.BaseStream) as DependencyObject;
Content = rootObject;
//...
|
|
l'attributo ThreadStatic indica che il valore di un campo statico è unico per ciascun thread, di seguito è riportato un esempio che mostra come utilizzare tale attributo e gli effetti esso che provoca.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading;
namespace UsingThreadStatic
{
class Program
{
[ThreadStatic]
static Int32 s_count = 0;
static Object s_synchro = new Object();
static void Main(string[] args)
{
for (int i = 0; i < 3; i++)
{
Thread thread = new Thread(() =>
{
while (true)
{
Console.WriteLine("Thread {0}, Count = {1}",
Thread.CurrentThread.Name, Interlocked.Increment(ref s_count));
Thread.Sleep(1000);
}
});
thread.Name = i.ToString();
thread.IsBackground = true;
thread.Start();
}
Console.Write("Press ENTER to close the host");
Console.ReadLine();
}
}
}
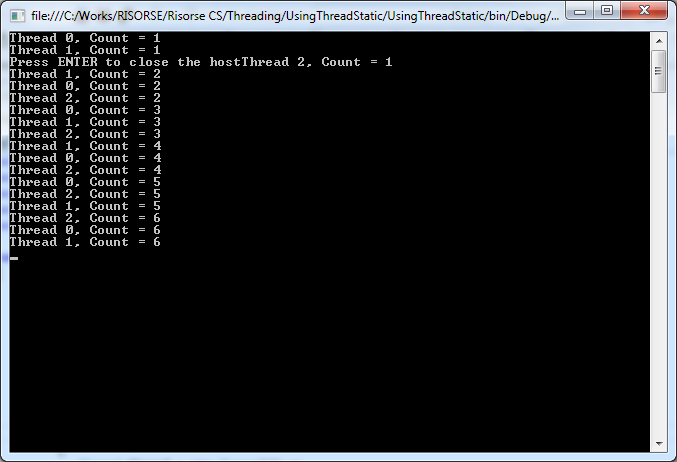
Come si può vedere dall'immagine riportata sopra la variabile s_count contiene un valore che è unico per thread, al contrario nell'immagine sotto si vede il risultato dell'applicazione eliminando l'attributo ThreadStatic:
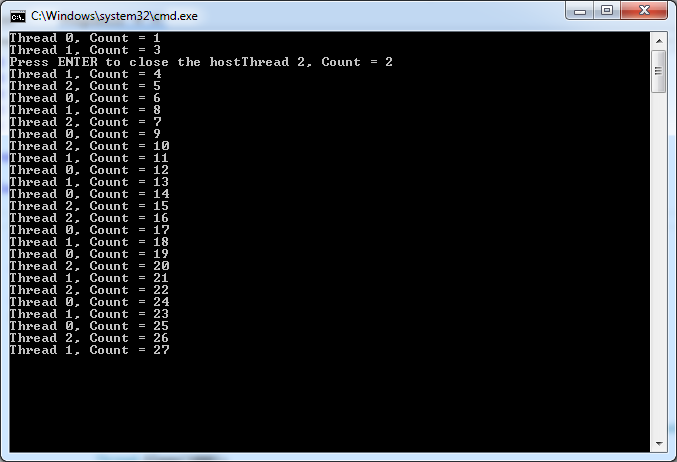
|
|
Per esecuzione posticipata si intende che la valutazione di un'espressione viene ritardata finché il relativo valore realizzato non risulta effettivamente necessario.
L'esecuzione posticipata può contribuire a migliorare notevolmente le prestazioni quando è necessario modificare grandi raccolte di dati, in particolare in programmi che contengono una serie di modifiche o query concatenate.
Nel migliore dei casi l'esecuzione posticipata consente di eseguire un'unica iterazione nella raccolta di origine.
L'esecuzione posticipata è supportata direttamente nel linguaggio C# tramite la parola chiave yield (sotto forma di istruzione yield-return) quando viene utilizzata all'interno di un blocco iteratore.
Tale iteratore deve restituire una raccolta di tipo IEnumerator o IEnumerator (o un tipo derivato).
// metodo che effettua la ricerca dell'elemento specificato all'interno
// di un array con esecuzione posticipata
public static IEnumerable<T> SearchDeferred<T>(this IEnumerable<T> array, T element)
{
foreach (T item in array)
{
if (item.Equals(element))
{
Console.WriteLine("Found item: {0}", element);
yield return item;
}
}
}
|
|
Un "Job Object" consente a gruppi di processi di essere gestiti come una singola unità.
Ad ogni Job Object può essere assegnato un nome, una protezione, i processi ad esso associati possono condividere oggetti e le operazioni eseguite su un job influenzano tutti i processi associati.
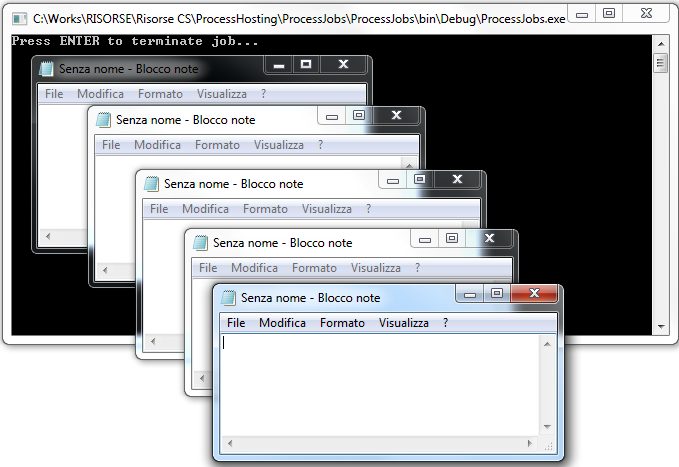
|
|
Esempio che illustra come creare istanze di componenti COM in .NET attraverso l'attributo ComImport
// MyCOM.idl : IDL source for MyCOM
//
// This file will be processed by the MIDL tool to
// produce the type library (MyCOM.tlb) and marshalling code.
import "oaidl.idl";
import "ocidl.idl";
[
object,
uuid(E16A9B85-A7B3-44DD-92FD-A18730260899),
dual,
nonextensible,
pointer_default(unique)
]
interface IMyClass : IDispatch{
[id(1)] HRESULT Initialize(void);
[propget, id(2)] HRESULT Prop1([out, retval] LONG* pVal);
[propput, id(2)] HRESULT Prop1([in] LONG newVal);
};
[
uuid(F1266AF0-1C30-4B9A-AFB0-C7E96CB1CCF1),
version(1.0),
]
library MyCOMLib
{
importlib("stdole2.tlb");
[
uuid(BE770998-A577-418D-9F41-592A11287623)
]
coclass MyClass
{
[default] interface IMyClass;
};
};
|
|
Questo breve esempio mostra come un'applicazione Windows Forms può ospitare un'altra applicazione (ad esempio notepad)
|